Haskell Course - Lesson 1 - Introduction and GHCi
Some courses start by explaining all the properties of Haskell. Others by dedicating entire lessons/chapters to the theory behind the language, Category theory (a branch of mathematics), lambda calculus (more maths), etc. Don't worry β I can hear you hyperventilate β we won't do that here. π
Those approaches have nothing wrong, but they are designed to teach people with previous knowledge of mathematics and computer science β which most don't have.
This course is geared to people that do NOT code The lecture will be written as if the person already knows basic computing skills (like installing programs and searching over the internet β π§πΌ will have a hard time, but they are welcome! π) but nothing about programming. And further explanations will be provided by clicking on highlights like
Ok. Are you ready? Let's go! π₯
GHCi (Glasgow Haskell Compiler Interactive)
A
Compiler
A compiler is a program that translates computer code written in one programming language (the source language) into another language (the target language). The name "compiler" is primarily used for programs that translate source code from a high-level programming language to a lower level language (e.g. assembly language, object code, or machine code) to create an executable program.
Program
A computer program is a sequence of instructions in a programming language that a computer can execute or interpret.
A computer program in its human-readable form is called source code. Source code needs another computer program (compiler or interpreter) to execute because computers can only execute their native machine instructions.
Programming language
A programming language is a formal language used by programmers (developers) to communicate instructions to computers. Usually, programming languages are written in plain text where each word, symbol, and number, has a pre-defined meaning for that specific language.
Example:

Intepreters
An interpreter is a computer program that directly executes instructions written in a programming or scripting language without requiring them previously to have been compiled into a machine language program.
The cool thing is that it doesn't matter if you have Windows, macOS, Linux, or whatever. Write Haskell, and the compiler will manage the rest. Cool right?
GHC (Glasgow Haskell Compiler) is a compiler and interactive environment for Haskell. Using GHC we can:
- Compile programs and execute them like any other app.
- Evaluate Haskell expressions on the fly using the interactive environment provided by the GHC (the GHCi).
For now, we'll use the GHCi. This interactive environment allows us to write code, hit enter, and get the result instantly! β‘οΈβ‘οΈ Allowing us to verify hypotheses faster than a 90' movie hacker.
The Terminal/Console/Shell
We'll use the GHCi through our terminal. The terminal is a text-only window through which we can interact with our computer. You write text, hit enter, stuff happen, and you get text as response (most of the time).
Terminal, shell, and command-line are technically different things. But many use those terms interchangeably β especially in programming tutorials. π So, keep in mind that if someone says "go to the command-line" or "open the shell," they talk about the terminal app π.
Here's how it looks:
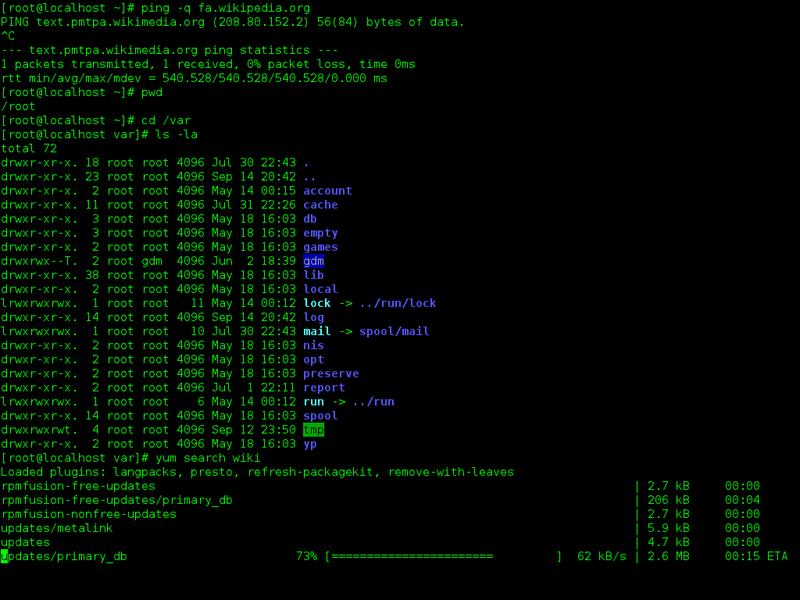
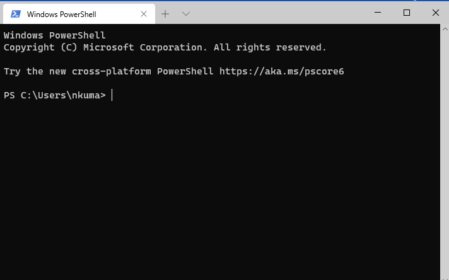
All operating systems have a Terminal app already installed.
We won't learn to use the terminal here because we'll only need a single
Terminal command
A command is a directive to a computer program to perform a specific task.
Examples
A command to change directory (cd) to the home directory:
cd /home
A command to list (ls) all files (-a) in the current directory:
ls -a
Installing GHCi
GHCi is usually installed with other tools (like Cabal, Stack, and a language server), but we don't need them for now, so we'll only install GHC. You can install the other tools later when you need them.
Each operating system has its own steps. I'll show you the most common ways. Select your OS (operating system) and follow the instructions:
Installing GHC in Linux
If you rock Linux, you know how this goes:
Ubuntu
sudo apt-get ghc
Fedora
sudo dnf install ghc
Other package-managers:
Follow the official documentation
Using distribution packages:
Installing GHC in macOS
Using Homebrew
If you already have Homebrew installed, open the Terminal and run this command:
brew install ghc
Download from the oficial website:
- Go to the official website and download GHC
- Make sure that you have the command line tools package of Xcode 4 or XCode 5 You can find Xcode at Apple developer portal.
Using GHCup (installer):
Execute the command provided here and follow the instructions.
Troubleshooting:
If you have any problems, Look up tutorials on YouTube
Using an installer:
- Download the installer from the official site
- Follow the instructions in the official documentation
Troubleshooting:
If you have any problems, Look up tutorials on YouTube
Let's test if everything is OK. Open your terminal, type ghci
and hit Enter. You should see a line saying your GHCi version and another line with Prelude>
waiting for you to write something.
The first thing that we'll learn is how to quit using GHCi. On Prelude>
type :q
and hit enter. It'll quit GHCi and return you to your terminal. To close your terminal, just close it like any other program.
Awesome! You already took your first steps to become a programmer! π We're ready to start our Haskell journey! π
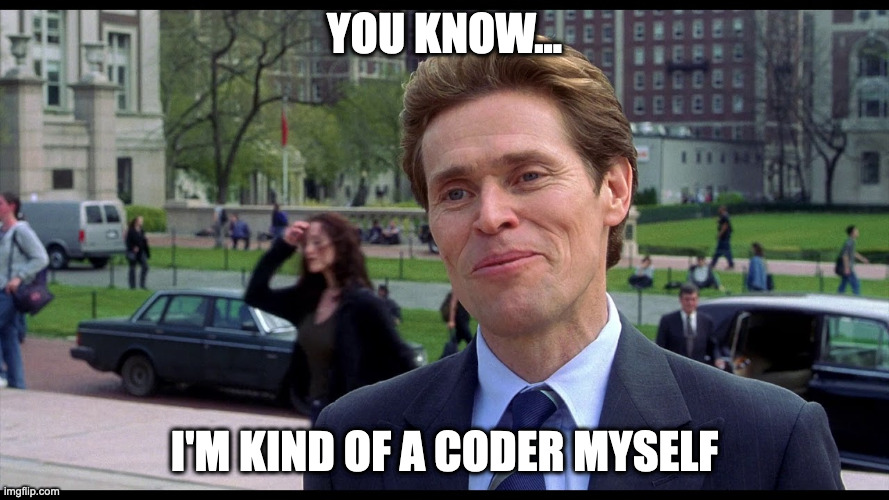
Homework
I'll provide some homework and the solution to the previous lesson's homework in each lesson. In this case, though, there isn't much to ask. So I'll give you some tasks that will help you level up your programming knowledge in general:
- Search and learn the difference between source code and machine code. (Searching and finding information is a crucial skill for programmers. We can't β and don't β remember everything about every technology we use. So, if you want to be a great coder, you have to be an expert searcher.)
- Search and learn the difference between a compiler and an interpreter.
- Familiarize yourself with the terminal: Search how to use the
cd
andls
commands.
Don't worry if you don't understand everything. If you "kind of" understand, that's good enough. Each time you encounter the concept, you'll gain a little bit more insight πͺ.
That's it! π In the next lesson, we'll learn about Haskell Types! π€© See you there!!
PD: If you find this course valuable, please share it so more people can learn! π